Man it's been a while since i've last posted and im really sorry for that. Anyway i've been starting on a 2nd Open Source Project I call Red Flag (name is meant to be a joke at the stereotype that Open Source is Socialist/Communist) which is going to be a Del.icio.us client for Mac OS X that offers more organizational and browsing abilities than Cocoalicious offers. I like Cocoalicious, I just want to do more with my bookmarks on a native Mac OS X Client. Plus school has been a bit more work than I thought at times, because as much as I love my Data Structures with Java course it can be a time consumer sometimes. And for those who took a look at my personal blog, no World of Warcraft hasn't drained huge amounts of time from me preventing me from doing this.. just so you know. Im doing great now.
Anyway onto the topic at hand. If you haven't heard of F-Script you really should take a look at it as it can help you in many situations. I must admit I haven't been using it for long, but already what i've seen has impressed me. I was introduced to F-Script at WWDC 06 and thought it was something interesting but didn't pick it up till about a month ago. First in a nutshell (quoting Wikipedia) F-Script is
F-Script is an object-oriented scripting programming language developed by Philippe Mougin. In a nutshell, F-Script is Smalltalk with support for Array programming.What does that mean? Well F-Script can do many things. You can use it to create small test apps on the fly, you can take control of your app at run time and browse the state of various objects without using Xcode, the debugger, special frameworks,etc. You can do various tests in F-Scripts stand alone console. Additionally F-Script is Open Source licenced under the BSD license. Syntax First off I should show you what's different between F-Script Syntax and regular Objective-C Braces... Objective-C
*color1 = [NSColor blueColor];F-Script
color1 := NSColor blueColorAs you can see F-Script doesn't use the Objective-C braces Strings F-Script using single quotes for strings instead of Objective-C's @"". Example Objective-C
NSString *string1 = [NSString stringWithString:@"This is a standard string"];F-Script
string1 := NSString stringWithString:'This is a standard string'Assignment As you've noticed above F-Script doesn't use a simple "=" for it's assignment operator it instead uses the colon equals ":=" ie Objective-C
color1 = [NSColor blueColor];F-Script
color1 := NSColor blueColorTypes Lastly you should have noticed that none of the F-Script examples had a type declaration on them. That's because everything in F-Script is of the type id which is to say everything is a generic object. Basic F-Script Components Well when your first starting out with F-Script there are 2 components your primarily concerned about.
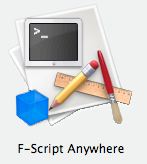
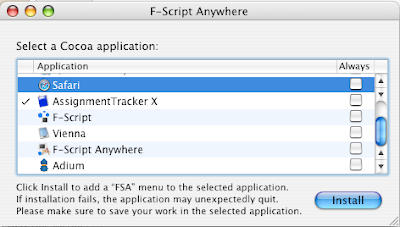
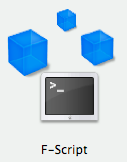
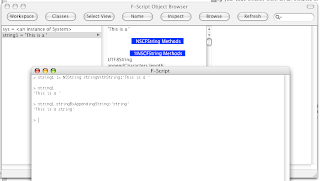
"F-SCRIPT CURRENCY CONVERTER (VERSION 1)" "Instantiate and configure a window" window := NSWindow alloc initWithContentRect:(125<>513 extent:383<>175) styleMask:NSTitledWindowMask + NSClosableWindowMask + NSMiniaturizableWindowMask backing:NSBackingStoreBuffered defer:NO. "Put the window onscreen" window orderFront:nil. "Give a title to the window" window setTitle:'Currency Converter'. "Instantiate a form object" form := NSForm alloc initWithFrame:(15<>70 extent:348<>85). "Put the form into the window" window contentView addSubview:form. "Configure the form" form addEntry:'Exchange Rate per $1'. form addEntry:'Dollars to Convert'. form addEntry:'Amount in Other Currency'. form setInterlineSpacing:9. form setAutosizesCells:YES. "Instantiate a decorative line and put it in the window" line := NSBox alloc initWithFrame:(15<>59 extent:353<>2). window contentView addSubview:line. "Instantiate a button, put it in the window and configure it" button := NSButton alloc initWithFrame:(247<>15 extent:90<>30). window contentView addSubview:button. button setBezelStyle:NSRoundedBezelStyle. button setTitle:'Convert'. button setKeyEquivalent:'\r'. "Create the script that will compute the currency conversion" conversionScript := [(form cellAtIndex:2) setStringValue:(form cellAtIndex:0) floatValue * (form cellAtIndex:1) floatValue]. "Make the script the target of the form. The script will be evaluated when the user presses Return" form setTarget:conversionScript. form setAction:#value. "Make the script the target of the button. The script will be evaluated when the user presses the button" button setTarget:conversionScript. button setAction:#value.What's great about this console is that is also comes with excellent code completion. My only complaint is that it doesn't filter on the fly like Xcode, but it's definitely a step up from other code completion systems out there.
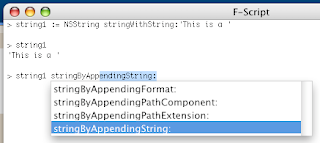
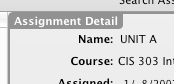
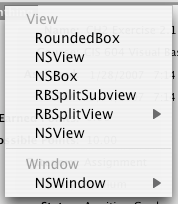
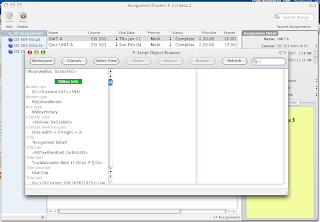
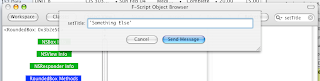
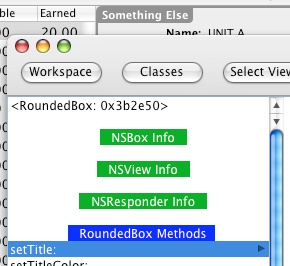
- We got a basic intro to what F-Script is
- We saw the basic differences between F-Scripts syntax and Objective-C's
- We learned basic information about F-Scipts 2 major components ( F-Script Anywhere and the F-Script console)
- We learned how to change the state of an object on the fly